JavaScript DOM - Code Exercises
- By David Abram
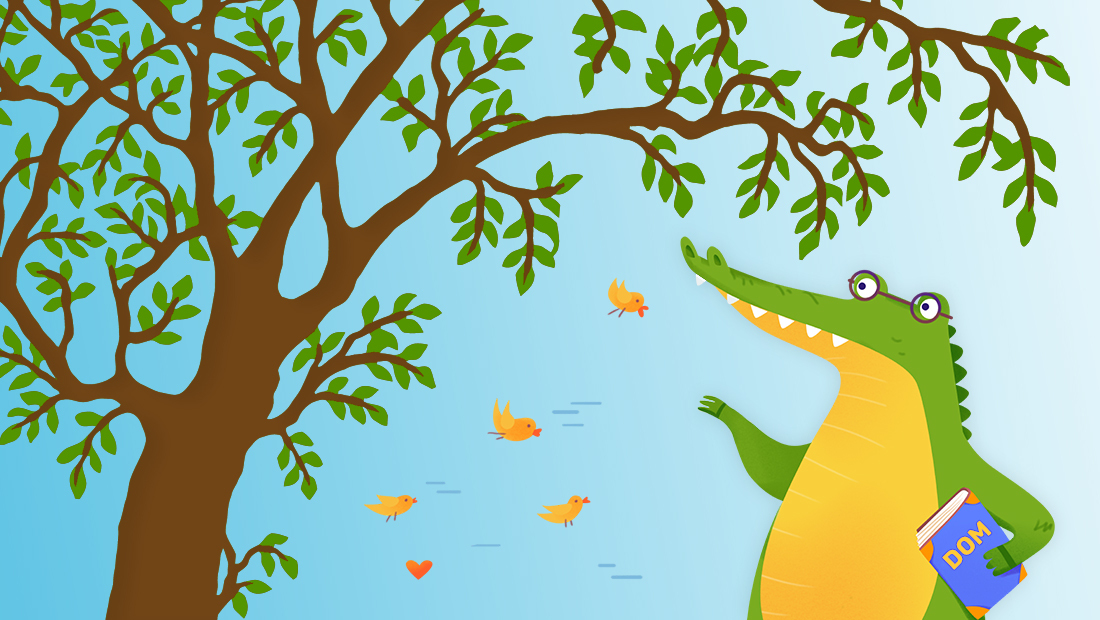
It’s really hard to test your programming knowledge after you have completed a tutorial or a lecture. We have prepared some exercises to help out beginner devs to solidify their understanding of the DOM. Every exercise has a brief description of the problem, starting code, links to relevant MDN docs, and expected results. Try to solve the problems without taking a peek at the solution.
If you need some additional help, you can check out our The DOM video from the #lockdown learning series or contact the author of the article directly.
The DOM or the Document Object Model of the page is created after the web page is loaded. It’s constructed as a tree of objects, and with it, JavaScript can access and change all the elements of an HTML document.
Content
Fruit list
You are given some names of different fruit and an unordered list html element; add a list item for each to the unordered list.
Helpful links
<ul></ul>
const fruitList = ["apple", "banana", "tomato"];
Result
<ul>
<li>apple</li>
<li>banana</li>
<li>tomato</li>
</ul>
Show Solution
const fruitList = ["apple", "banana", "tomato"];
const ulElement = document.querySelector("ul");
fruitList.forEach((fruit) => {
const itemElement = document.createElement("li");
itemElement.textContent = fruit;
ulElement.appendChild(itemElement);
});
This code creates a constant array fruitList
with three elements, apple
, banana
, and tomato
. It then selects an unordered list element from the HTML document using document.querySelector('ul')
.
Using the forEach
method on the fruitList
array, the code creates a new list item element for each fruit in the list, sets its text content to the name of the fruit, and appends it as a child to the unordered list element. This will dynamically add the list of fruits as list items in the HTML document.
CroCoder logo
Add the CroCoder logo (image element) as a child element to the existing div element.
Helpful links
<div></div>
const imageSrc = "https://crocoder.dev/icon.png";
Result
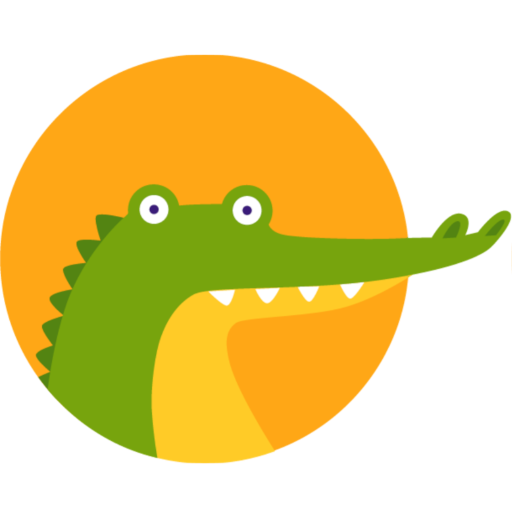
Show Solution
const imageSrc = "https://crocoder.dev/icon.png";
const divElement = document.querySelector("div");
const imgElement = document.createElement("img");
imgElement.src = imageSrc;
divElement.appendChild(imgElement);
This code creates a constant imageSrc
with a string value of an image URL. It then selects a div element from the HTML document using document.querySelector('div')
.
The code then creates an img
element using document.createElement('img')
and sets its src
attribute to the value of imageSrc
. Finally, the code appends the img
element as a child to the div
element using divElement.appendChild(imgElement)
. This will dynamically add the image from the URL specified in imageSrc
as an element inside the div
in the HTML document.
Change first and last item from an unordered list
Change the text in the first and the last list item element in every unordered list element on this page. Change it to 'first'
and 'last'
.
Helpful links
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>
<ul>
<li>a</li>
<li>b</li>
<li>c</li>
</ul>
<ul>
<li>👻</li>
<li>👽</li>
<li>🦁</li>
</ul>
Result
<div>
<ul>
<li>first</li>
<li>2</li>
<li>last</li>
</ul>
<ul>
<li>first</li>
<li>b</li>
<li>last</li>
</ul>
<ul>
<li>first</li>
<li>👽</li>
<li>last</li>
</ul>
</div>
Show Solution
const firstLis = [...document.querySelectorAll("ul > li:first-child")];
const lastLis = [...document.querySelectorAll("ul > li:last-child")];
firstLis.forEach((li) => (li.textContent = "first"));
lastLis.forEach((li) => (li.textContent = "last"));
This code is using JavaScript to select and manipulate HTML elements. The first line uses the querySelectorAll
method to find all the first children li
elements within ul
elements and spread them into an array firstLis
.
The second line does the same for all the last children li
elements within ul
elements and spread them into an array lastLis
. Then, the forEach
method is used to iterate over both arrays and modify the textContent
property of each li
element to be “first” for the elements in firstLis
and “last” for the elements in lastLis
.
List of items
You are building a web page that displays a list of items. The user should be able to add new items to the list and edit the text of the items. Your task is to use the DOM API to create the necessary elements, add event listeners to them, and manipulate the elements as the user interacts with the page.
Helpful links
<div id="list-app">
<div>
Result
- Map, filter, reduce
- The DOM
- Callbacks
Show Solution
const app = document.getElementById("list-app");
// Create the necessary elements
const list = document.createElement("ul");
const input = document.createElement("input");
input.setAttribute("type", "text");
const addButton = document.createElement("button");
addButton.innerHTML = "Create New";
// Add event listeners to the elements
addButton.addEventListener("click", function() {
const newItem = document.createElement("li");
newItem.innerHTML = 'New item';
list.appendChild(newItem);
input.value = "";
});
list.addEventListener("click", function(event) {
if (event.target.tagName === "li") {
const text = prompt("Enter new text:");
event.target.innerHTML = text;
}
});
app.appendChild(input);
app.appendChild(addButton);
In this code snippet, we created three elements: list
which is an unordered list, input
which is a text input, and addButton
which is a button element.
We use the setAttribute
method to set the type of the input to “text”. Then, we use the addEventListener
method to add event listeners to the elements. When the user clicks the add button, the function inside the event listener will create a new li
element, set its text to the value of the input, and append it to the list. When the user clicks on an item in the list, the function inside the event listener will prompt the user for new text and set the text of the item to the new text.
Finally, we use the appendChild
method to add the elements to the body of the web page.
Please note that this is just a simplified example. You might want to add some error handling, validation, and more features when building your own application.
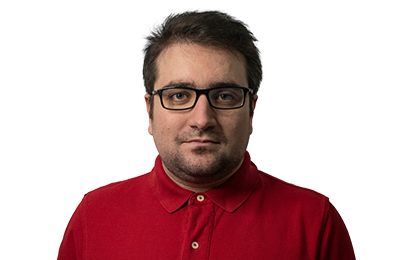
Spends his time untangling software architectures and doing DevOps. Likes to build stuff.
Connect with David on X and LinkedIn. You can also Book a meeting with David.